Today, everyone is building AI-featured applications. There are many ways to utilize Large Language Models in your app, ranging from simple API calls to using frameworks. The next question is which technology to choose for building the frontend of your application. Traditionally, AI frameworks are built using Python, while JavaScript is a popular choice for web frameworks. After completing several projects, we've settled on a setup that works well for us.
For AI, we adopt an agent-based approach that elevates the capabilities of modern LLMs to the next level. This approach is quite flexible, allowing for greater control over the LLM when needed or enabling it to choose how to solve a problem independently. Several good frameworks for building agentic applications are available on the market, such as CrewAI, AutoGen, PydanticAI, and LangGraph. In my opinion, LangGraph is the most sophisticated option, offering exceptional flexibility, excellent tools, and all the necessary features to develop AI applications.
For the frontend, we prefer Next.js. It is an excellent full-stack framework that provides everything needed to build modern web applications.
Integrating LangGraph with Next.js is not straightforward. The first issue is that LangGraph is a Python framework, while Next.js is a JavaScript framework. Therefore, we need an API on top of LangGraph to use it with Next.js. The next challenge is synchronizing LangGraph’s state with the frontend application and making it convenient for developers to work with.
In this blog post, I will describe the technical details of the solution we made. Alternatively, you can check out the Git repository right away!
Create AI agent with LangGraph
What is LangGraph
LangGraph is a library for building stateful applications with LLMs. It can be used to create AI agent and multi-agent systems or to establish predefined code paths around LLM calls. The framework offers low-level control over flow execution, providing flexibility in its usage. You define your graph where nodes are essentially functions containing your custom code. Then, you establish edges between those nodes. The graph has a state, which is simply a dictionary of key-value pairs. This state updates after each node execution.
LangGraph has a plenty cool features:
- Persistence. It saves the state of the graph before and after node execution as a checkpoint. You can replay or fork graph execution from a specific checkpoint. If you are creating a simple chatbot app, there is no need to develop save message logic; it is already handled in LangGraph.
- Human-in-the-loop. It is one of the crucial features in AI agent development. Graph execution can be interrupted and resumed after user input. Asking users for confirmation before executing important actions is a good practice. For instance, when building a booking service, the agent finds hotel suites that meet user requirements, then asks for user confirmation to proceed. Only after receiving a positive response does it call the booking API to make a reservation.
- Streaming. The nature of LLMs is that they do not answer immediately, especially when generating long outputs. One common UX pattern for AI applications, particularly chatbots, is streaming. This means displaying part of the response as soon as it becomes available.
- Sub graphs. LangGraph allows the use of other graphs as nodes within the graph. This powerful approach enables feature reuse. Imagine you have a RAG agent app for searching information in your documents or on the web. You can easily integrate this AI agent into a new research agent by simply adding it as a node to the graph.
- Tooling. Debugging LLM applications is not an easy task. That's why having tools for evaluation and monitoring is essential. LangGraph Studio is a desktop application that allows developers to visualize the graph app and run it from a specific point. LangSmith is a monitoring and evaluation platform for your AI applications.
- Community. LangGraph is built on LangChain, one of the most popular frameworks for working with LLMs. There is a vast array of components created by the community that can be used in LangGraph.
How to build an AI agent
There are many definitions of AI agents. Personally, I prefer this one: an agent is a system that uses AI to determine the control flow of an application. As developers, we describe how the system should behave and what tools it has access to. In real-life cases, it is more complex, but we will examine a simplified example.
Let’s build an assistant which can tell realtime weather and create reminders. We will have the following architecture: LLM with two tools: weather and reminder.
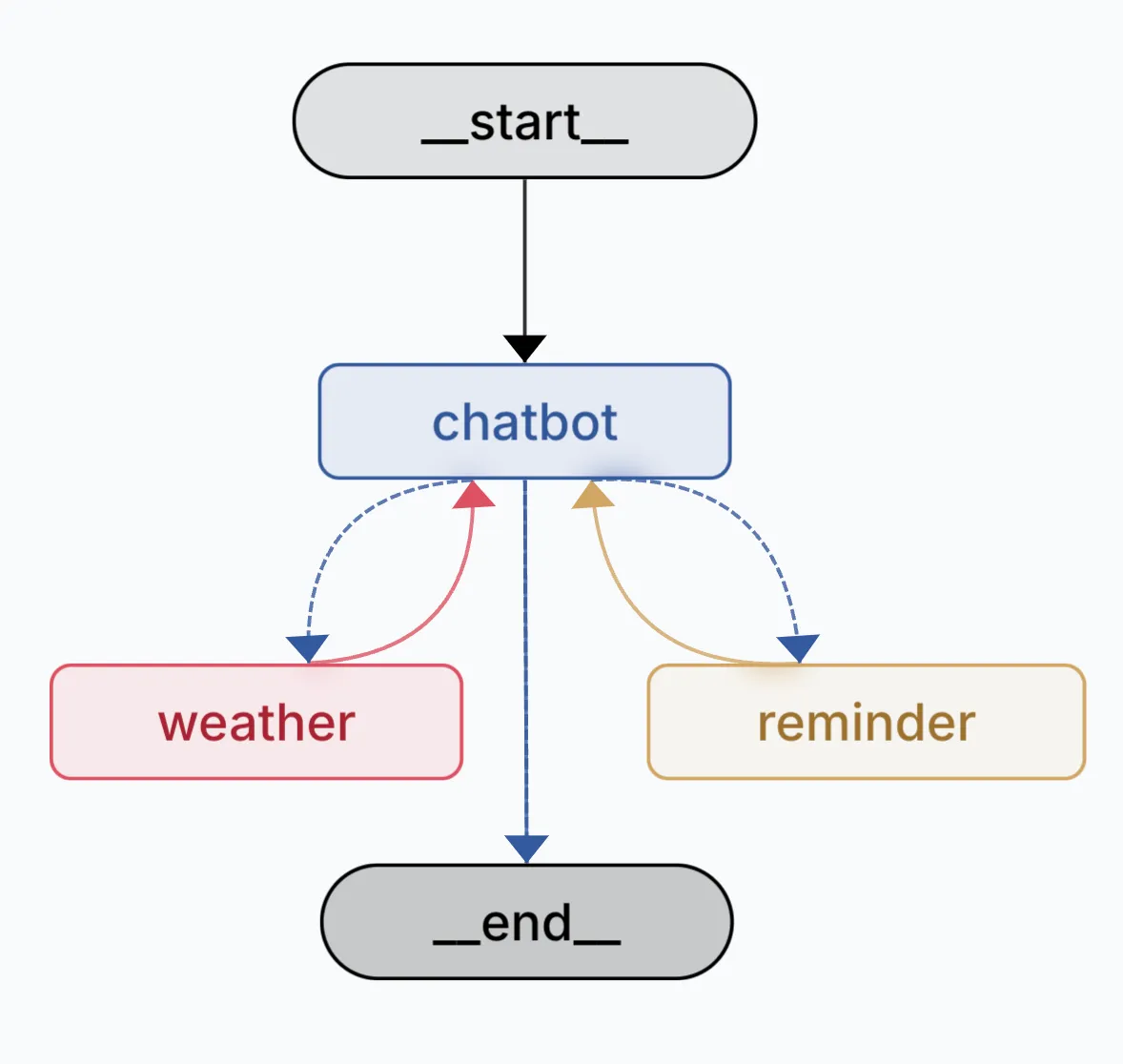
The definition of tools is quite straightforward. We describe what a tool does and its input parameters. Don’t be confused by the return statement on the screen; it is only for testing purposes. In real life, the weather tool should use a web search service or a weather API. And the reminder tool should create reminders in the database.
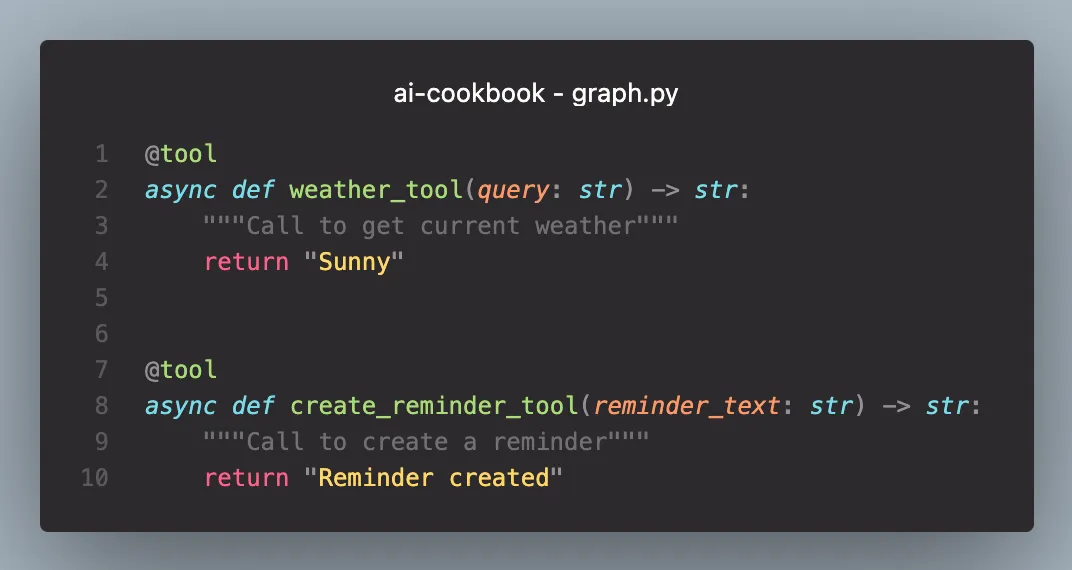
LLM decides when and which tools to use based on user input. For example, if a user asks, “What is the weather in LA and SF?”, LLM understands that it should call the weather tool twice: first to check the weather in Los Angeles and then to check the weather in San Francisco.
But it can involve more complex scenarios. Imagine a user asking the assistant, “What is the forecast for this weekend? If it is rainy, remind me to buy a raincoat.” The flow will look something like this:
- The LLM will ask the user about their location
- After the user provides their location, it will call the weather tool
- If the weather tool indicates rainy weather, it will then call a reminder tool to create a reminder.
The entire program flow is determined by AI. Awesome, isn’t it?
Create agent API
Our LangGraph AI agent is written in Python, and we cannot use it directly in our Next.js application. We need an API to allow client apps to utilize it. For this purpose, we used the FastAPI framework.
There are several endpoints client application might need:
- POST /agent - main endpoint to run or resume agent execution.
- POST /agent/stop - initiates agent stop.
- GET /history - returns the list of graph checkpoints. This list is used to restore the graph execution runs for display on the UI.
Create web application with Next.js
To build our end-user web app, we are using Next.js. This popular React-based framework offers a comprehensive solution for creating modern web applications. At Akveo, we utilize Next.js to deliver solutions to our clients quickly. We also build our internal apps with it. As a full-stack framework, it includes all the necessary built-in features, allowing you to start building without spending time on configuration.
Next.js features
Here are the advantages of the framework:
- Server-Side Rendering (SSR): Improves SEO and performance by rendering pages on the server before sending them to the client.
- Static Site Generation (SSG): Allows pre-rendering of pages at build time, resulting in faster load times.
- API Routes: Simplifies backend integration by allowing you to create API endpoints within the same application.
- Automatic Code Splitting: Only loads the necessary JavaScript for the page being accessed, enhancing performance.
- File-Based Routing: Simplifies routing by using the file system, making it easy to create and manage routes.
- Built-in CSS and Sass Support: Offers support for global and modular CSS, as well as Sass, without additional configuration.
- Image Optimization: Automatically optimizes images for better performance and user experience.
- Rich Ecosystem: Integrates well with React and has a large community and ecosystem of plugins and tools.
Agent integration
First, let's explore what the execution of the graph (our AI agent) means. LangGraph creates checkpoints before app execution, before each graph node, and after app execution. A checkpoint is essentially a snapshot of the graph's state, indicating which node or nodes will be executed next. Here is a screenshot from LangGraph Studio illustrating an execution example. In the right panel, the grey rectangles represent the checkpoints, while the circles indicate the nodes that were executed. You can inspect both the state of the checkpoint and the individual nodes.

To gain full control and access graph execution data, we need to replicate this architecture in the client app. The logic is encapsulated in the useLangGraphAgent
hook, which calls the AI service API and syncs the agent state on the client side.
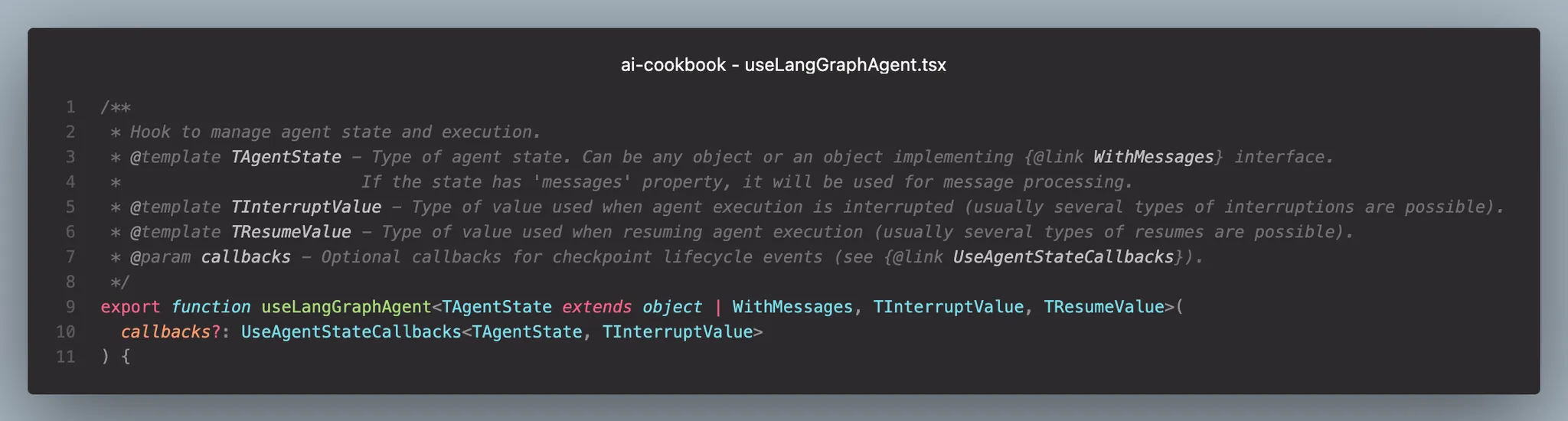
Using the exposed methods and objects from the hook, we can build any application logic and user interface based on them. Here is the description.
Properties:
- status: indicates the agent's execution status
- appCheckpoints: a list of graph checkpoints and nodes with their states
Methods:
- run: executes the agent with the provided state
- resume: continues agent execution after human interaction
- restore: retrieves the checkpoint history of a specific agent thread
- replay: re-executes the agent from a checkpoint
- fork: creates a fork of the checkpoint with a custom state and runs the agent
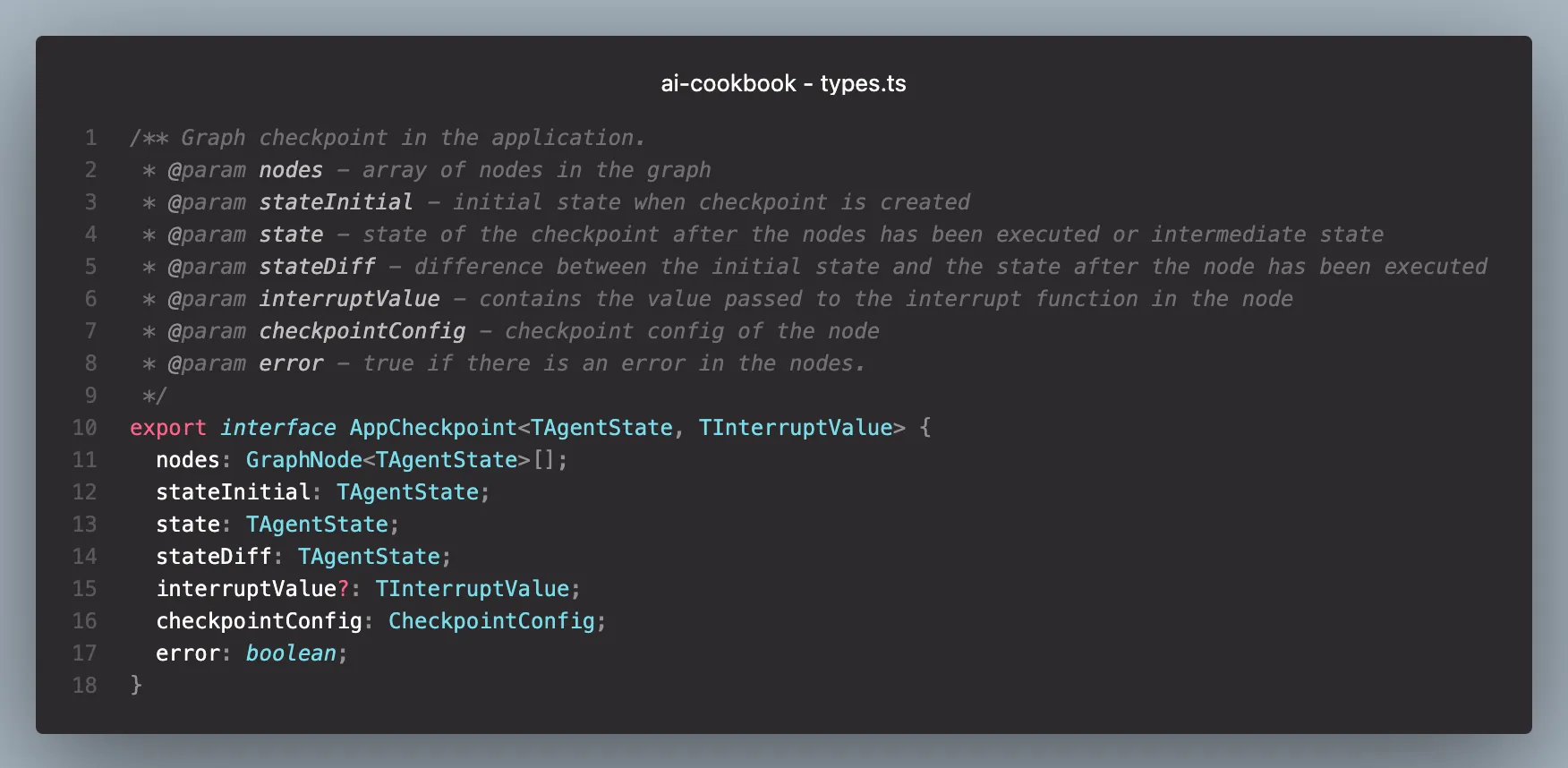
appCheckpoint
is the foundation of our app logic. Let’s explore its properties.
- nodes: the list of nodes executed in this checkpoint.
- stateInitial, state, stateDiff: these store the checkpoint state before and after node execution, along with a state difference for convenience.
- interruptValue: this value is used in human-in-the-loop cases. It holds the interruption value from the graph, such as a prompt for user confirmation.
- checkpointConfig: configuration required for graph replies or forks.
- error: indicates whether an error occurred in one of the child nodes.
Building the client app
The final step is to build a user interface for interacting with our AI agent. The most common user experience for communicating with AI is through a chat. When the user submits a new message, we call the run
method of the useLangGraphAgent
hook. While the agent is executing, new data streams from the AI service to the client app, and the appCheckpoints
array is populated. The only remaining task is to render chat messages based on the appCheckpoints
array.
We iterate over the checkpoints array and then over each node within a checkpoint. Based on the node name, we select the appropriate React component to render and pass only its relevant piece of state to the component.
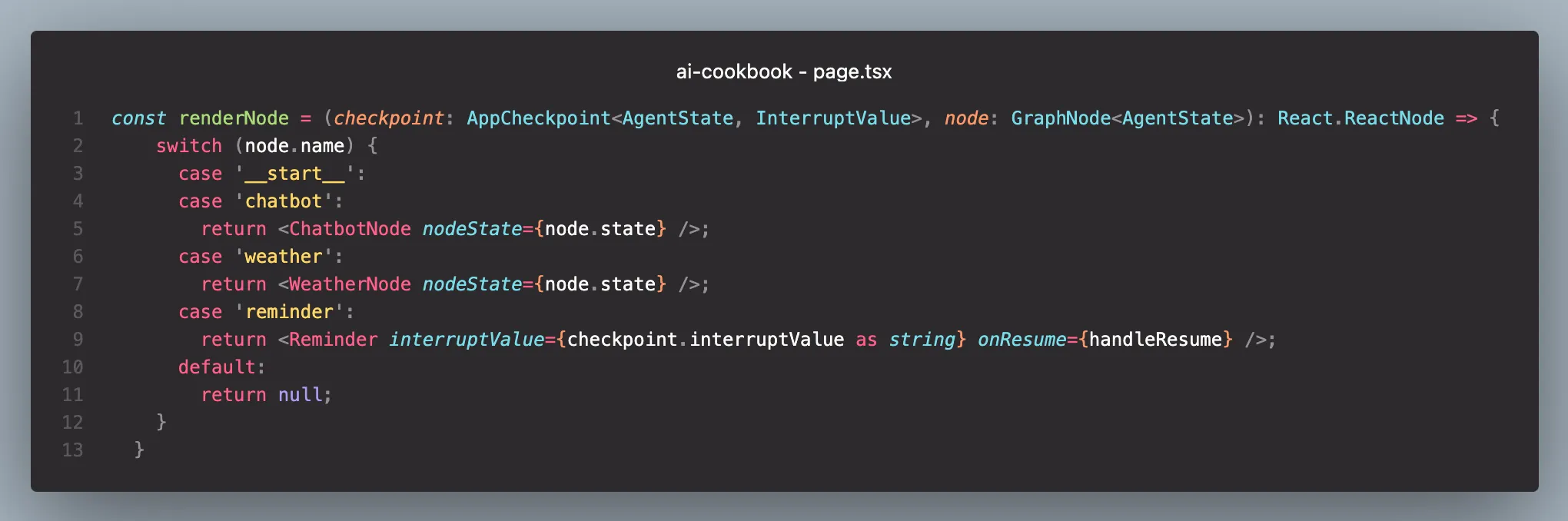
For a chatbot node, we use the ChatbotNode
component, which renders messages from either humans or AI.

However, things become interesting when the agent path leads to other nodes. In the weather node, we can display not just a text message but also a visually appealing animated weather widget.

When the app flow reaches the reminder node, the agent's execution is interrupted, and we wait for user feedback. In this case, we can render a card with confirmation text and possible actions.
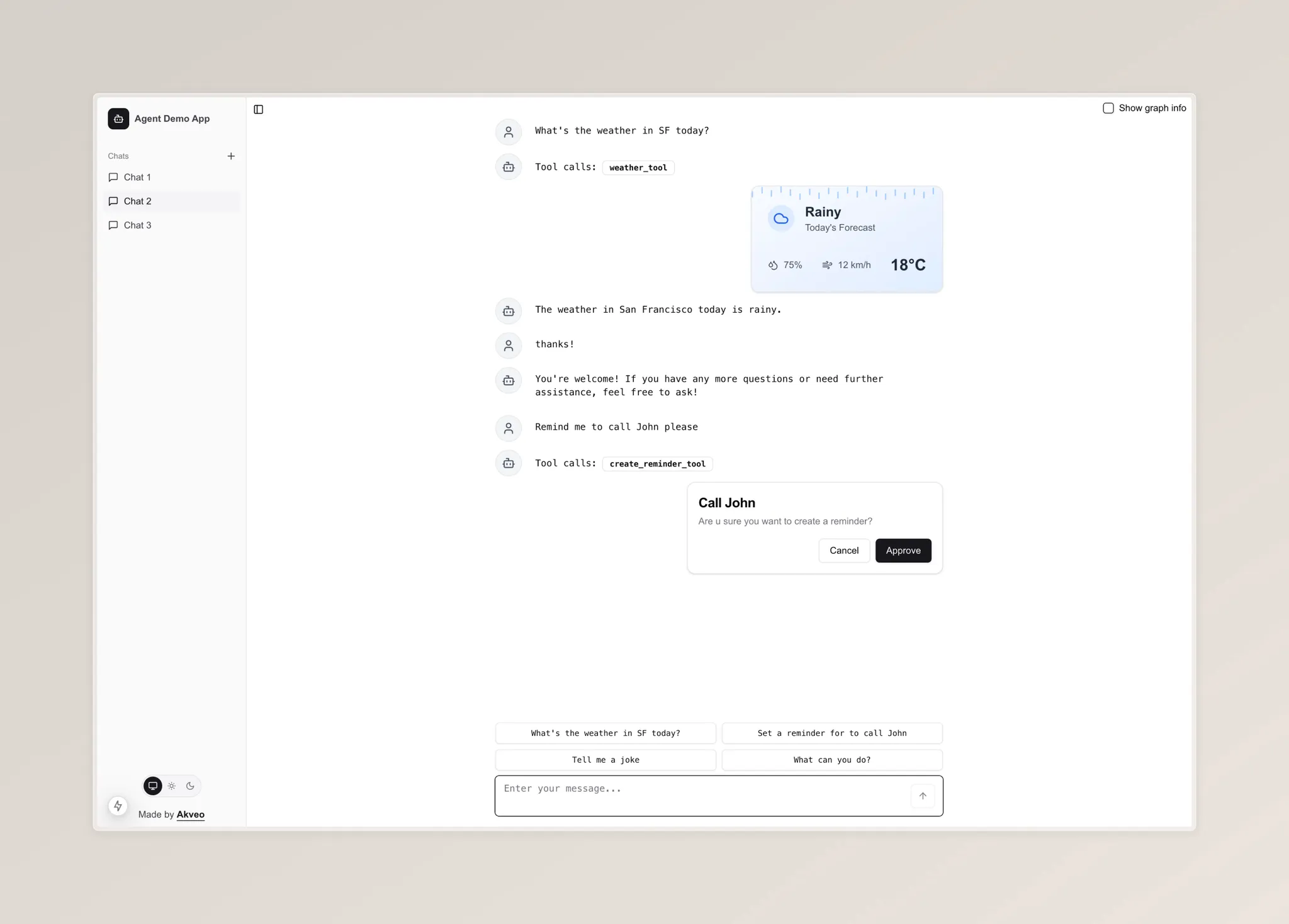
Another feature worth mentioning is that LangGraph has a built-in persistence layer, so we do not need to implement the logic for saving chat messages from scratch. Each agent execution is saved to its own thread. We simply need to call the restore
method of the useLangGraphAgent
hook with the threadId
parameter. This will invoke the AI service and populate the appCheckpoints array.
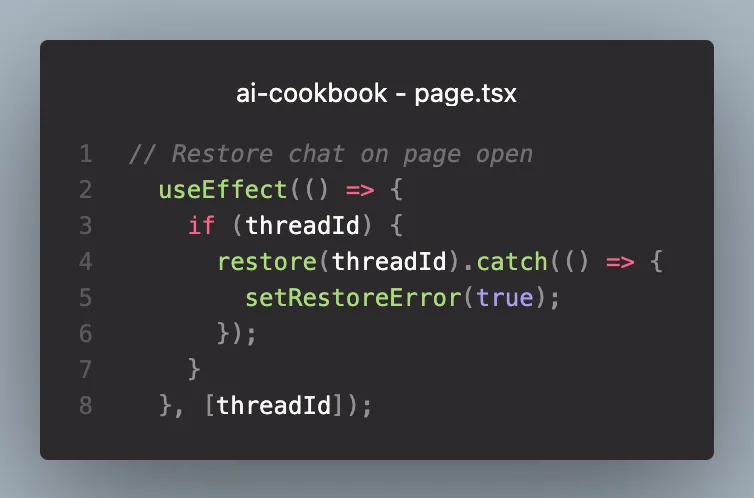
Summary
We created an AI agent using LangGraph and built a user interface for it with Next.js. I hope this inspires you to develop your own applications with AI features. Simply clone the akveo/ai-cookbook repository; it will serve as a great starting point for your ideas.
Streamlining Gifting Marketplace Operations with Retool
Afloat, a gifting marketplace, needed custom dashboards to streamline order management, delivery tracking, and reporting while integrating with Shopify and external APIs.
The solution:
We built two Retool-based dashboards:
- A Retail Partner Dashboard embedded into Shopify for managing orders and store performance.
- An Admin Dashboard for handling deliveries and partner data.
Both dashboards included real-time integration with Afloat's Backend and APIs for accurate, up-to-date data and scalability.
The result: enhanced efficiency, error-free real-time data, and scalable dashboards for high-order volumes.
Billing Automation for a SaaS Company with Low-Code
Our client needed a robust billing solution to manage hierarchical licenses, ensure compliance, and automate invoicing for streamlined operations.
The solution:
We developed a Retool-based application that supports multi-tiered licenses, automates invoicing workflows, and integrates seamlessly with CRM and accounting platforms to enhance financial data management.
The result:
- Achieved 100% adherence to licensing agreements, mitigating penalties.
- Automated invoicing and workflows reduced manual effort significantly.
- Dashboards and reports improved decision-making and operational visibility.
Retool Dashboards with HubSpot Integration
Our client needed a centralized tool to aggregate account and contact activity, improving visibility and decision-making for the sales team.
The solution
We built a Retool application integrated with HubSpot, QuickMail, and Clay.com. The app features dashboards for sorting, filtering, and detailed views of companies, contacts, and deals, along with real-time notifications and bidirectional data syncing.
The result
- MVP in 50 hours: Delivered a functional application in just 50 hours.
- Smarter decisions: Enabled data-driven insights for strategic planning.
- Streamlined operations: Reduced manual tasks with automation and real-time updates.
Lead Generation Tool to Reduce Manual Work
Our client, Afore Capital, a venture capital firm focused on pre-seed investments, aimed to automate their lead generation processes but struggled with existing out-of-the-box solutions. To tackle this challenge, they sought assistance from our team of Akveo Retool experts.
The scope of work
The client needed a tailored solution to log and track inbound deals effectively. They required an application that could facilitate the addition, viewing, and editing of company and founder information, ensuring data integrity and preventing duplicates. Additionally, Afore Capital aimed to integrate external tools like PhantomBuster and LinkedIn to streamline data collection.
The result
By developing a custom Retool application, we streamlined the lead generation process, significantly reducing manual data entry. The application enabled employees to manage inbound deals efficiently while automated workflows for email parsing, notifications, and dynamic reporting enhanced operational efficiency. This allowed Afore Capital's team to focus more on building relationships with potential founders rather than on administrative tasks.
Retool CMS Application for EdTech Startup
Our client, CutTime, a leading fine arts education management platform, needed a scalable CMS application to improve vendor product management and user experience.
The scope of work
We developed a Retool application that allows vendors to easily upload and manage product listings, handle inventory, and set shipping options. The challenge was to integrate the app with the client’s system, enabling smooth authentication and product management for program directors.
The result
Our solution streamlined product management, reducing manual work for vendors, and significantly improving operational efficiency.
Building Reconciliation Tool for e-commerce company
Our client was in need of streamlining and simplifying its monthly accounting reconciliation process – preferably automatically. But with a lack of time and low budget for a custom build, development of a comprehensive software wasn’t in the picture. After going through the case and customer’s needs, we decided to implement Retool. And that was the right choice.
The scope of work
Our team developed a custom reconciliation tool designed specifically for the needs of high-volume transaction environments. It automated the processes and provided a comprehensive dashboard for monitoring discrepancies and anomalies in real-time.
The implementation of Retool significantly reduced manual effort, as well as fostered a more efficient and time-saving reconciliation process.
Creating Retool Mobile App for a Wine Seller
A leading spirits and wine seller in Europe required the development of an internal mobile app for private client managers and administrators. The project was supposed to be done in 1,5 months. Considering urgency and the scope of work, our developers decided to use Retool for swift and effective development.
The scope of work
Our developers built a mobile application tailored to the needs of the company's sales force: with a comprehensive overview of client interactions, facilitated order processing, and enabled access to sales history and performance metrics. It was user-friendly, with real-time updates, seamlessly integrated with existing customer databases.
The result? Increase in productivity of the sales team and improved decision-making process. But most importantly, positive feedback from the customers themselves.
Developing PoC with Low Code for a Tour Operator
To efficiently gather, centralize, and manage data is a challenge for any tour operator. Our client was not an exception. The company was seeking to get an internal software that will source information from third-party APIs and automate the travel itinerary creation process. Preferably, cost- and user-friendly tool.
The scope of work
Our experts ensured the client that all the requirements could be covered by Retool. And just in 40 hours a new software was launched. The tool had a flexible and easy-to-use interface with user authentication and an access management system panel – all the company needed. At the end, Retool was considered the main tool to replace the existing system.
Testing New Generation of Lead Management Tool with Retool
Our client, a venture fund, had challenges with managing lead generation and client acquisition. As the company grew, it aimed to attract more clients and scale faster, as well as automate the processes to save time, improve efficiency and minimize human error. The idea was to craft an internal lead generation tool that will cover all the needs. We’ve agreed that Retool will be a perfect tool for this.
The scope of work
The project initially began as a proof of concept, but soon enough, with each new feature delivered, the company experienced increased engagement and value.
We developed a web tool that integrates seamlessly with Phantombuster for data extraction and LinkedIn for social outreach. Now, the company has a platform that elevates the efficiency of their lead generation activities and provides deep insights into potential client bases.
Building an Advanced Admin Portal for Streamlined Operations
Confronted with the need for more sophisticated internal tools, an owner of IP Licensing marketplace turned to Retool to utilize its administrative functions. The primary goal was to construct an advanced admin portal that could support complex, multi-layered processes efficiently.
The scope of work
Our client needed help with updating filters and tables for its internal platform. In just 30 hours we've been able to update and create about 6 pages. Following features were introduced: add complex filtering and search, delete records, styling application with custom CSS.
Together, we have increased performance on most heavy pages and fixed circular dependency issues.
Creating MVP Dashboard for Google Cloud Users
Facing the challenge of unoptimized cloud resource management, a technology firm working with Google Cloud users was looking for a solution to make its operations more efficient. The main idea of the project was to create an MVP for e-commerce shops to test some client hypotheses. Traditional cloud management tools fell short.
The scope of work
Determined to break through limitations, our team of developers turned Retool. We decided to craft an MVP Dashboard specifically for Google Cloud users. This wasn't just about bringing data into view; but about reshaping how teams interact with their cloud environment.
We designed a dashboard that turned complex cloud data into a clear, strategic asset thanks to comprehensive analytics, tailored metrics, and an intuitive interface, that Retool provides. As the results, an increase in operational efficiency, significant improvement in cost management and resource optimization.
Elevating CRM with Custom HubSpot Sales Dashboard
Our other client, a SaaS startup, that offers collaborative tools for design and engineering teams, was on a quest to supercharge their sales efforts. Traditional CRM systems were limited and not customizable enough. The company sought a solution that could tailor HubSpot to their workflow and analytics needs.
The scope of work
Charged with the task of going beyond standard CRM functions, our team turned to Retool. We wanted to redefine how sales teams interact with their CRM.
By integrating advanced analytics, custom metrics, and a user-friendly interface, our developers provided a solution that transformed data into a strategic asset.
In 40 hours, three informative dashboards were developed, containing the most sensitive data related to sales activities. These dashboards enable our customer to analyze sales and lead generation performance from a different perspective and establish the appropriate KPIs.
Building a PDF Editor with Low-Code
Our client, a leading digital credential IT startup, needed a lot of internal processes to be optimized. But the experience with low-code tools wasn’t sufficient. That’s why the company decided to hire professionals. And our team of developers joined the project.
The scope of work
The client has a program that designs and prints custom badges for customers. The badges need to be “mail-merged” with a person’s info and turned into a PDF to print. But what is the best way to do it?
Our developers decided to use Retool as a core tool. Using custom components and JavaScript, we developed a program that reduced employees' time for designing, putting the data, verifying, and printing PDF badges in one application.
As a result, the new approach significantly reduces the time required by the internal team to organize all the necessary staff for the conference, including badge creation.